Александр Степанов - РУКОВОДСТВО ПО СТАНДАРТНОЙ БИБЛИОТЕКЕ ШАБЛОНОВ (STL) Страница 19
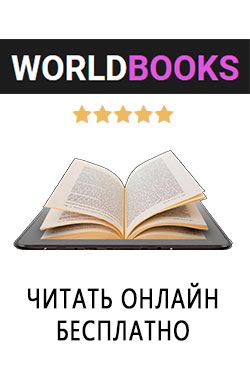
- Категория: Компьютеры и Интернет / Программирование
- Автор: Александр Степанов
- Год выпуска: -
- ISBN: нет данных
- Издательство: -
- Страниц: 30
- Добавлено: 2019-05-29 11:00:25
Александр Степанов - РУКОВОДСТВО ПО СТАНДАРТНОЙ БИБЛИОТЕКЕ ШАБЛОНОВ (STL) краткое содержание
Прочтите описание перед тем, как прочитать онлайн книгу «Александр Степанов - РУКОВОДСТВО ПО СТАНДАРТНОЙ БИБЛИОТЕКЕ ШАБЛОНОВ (STL)» бесплатно полную версию:Александр Степанов - РУКОВОДСТВО ПО СТАНДАРТНОЙ БИБЛИОТЕКЕ ШАБЛОНОВ (STL) читать онлайн бесплатно
const unsigned nameSize = sizeof(names)/sizeof(names[0]);
vector‹char*› v1(nameSize);
for (int i = 0; i ‹ v1.size(); i++) {
v1[i] = names[i];
}
vector‹char*› v2(2);
v2[0] = "foo";
v2[1] = "bar";
sort(v1.begin(), v1.end(), compare_strings);
sort(v2.begin(), v2.end(), compare_strings);
bool inc = includes(v1.begin(), v1.end(), v2.begin(), v2.end(), compare_strings);
if (inc) cout ‹‹ "v1 includes v2" ‹‹ endl;
else cout ‹‹ "v1 does not include v2" ‹‹ endl;
v2[0] = "Brett";
v2[1] = "Todd";
inc = includes(v1.begin(), v1.end(), v2.begin(), v2.end(), compare_strings);
if (inc) cout ‹‹ "v1 includes v2" ‹‹ endl;
else cout ‹‹ "v1 does not include v2" ‹‹ endl;
return 0;
}
search1.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
typedef vector‹int› IntVec;
IntVec v1(10);
iota(v1.begin(), v1.end(), 0);
IntVec v2(3);
iota(v2.begin(), v2.end(), 50);
ostream_iterator‹int› iter(cout, " ");
cout ‹‹ "v1: ";
copy(v1.begin(), v1.end(), iter);
cout ‹‹ endl;
cout ‹‹ "v2: ";
copy(v2.begin(), v2.end(), iter);
cout ‹‹ endl;
IntVec::iterator location;
location = search(v1.begin(), v1.end(), v2.begin(), v2.end());
if (location == v1.end()) cout ‹‹ "v2 not contained in v1" ‹‹ endl;
else cout ‹‹ "Found v2 in v1 at offset: " ‹‹ location - v1.begin() ‹‹ endl;
iota(v2.begin(), v2.end(), 4);
cout ‹‹ "v1: ";
copy(v1.begin(), v1.end(), iter);
cout ‹‹ endl;
cout ‹‹ "v2: ";
copy(v2.begin(), v2.end(), iter);
cout ‹‹ endl;
location = search(v1.begin(), v1.end(), v2.begin(), v2.end());
if (location == v1.end()) cout ‹‹ "v2 not contained in v1" ‹‹ endl;
else cout ‹‹ "Found v2 in v1 at offset: " ‹‹ location - v1.begin() ‹‹ endl;
return 0;
}
istmit2.cpp
#include ‹iostream.h›
#include ‹fstream.h›
#include ‹stl.h›
typedef vector‹char› Line;
void printLine(const Line* line_) {
vector‹char›::const_iterator i;
for (i = line_-›begin(); i!= line_-›end(); i++) cout ‹‹ *i;
cout ‹‹ endl;
}
int main() {
Line buffer;
vector‹Line*› lines;
ifstream s("data.txt");
s.unsetf(ios::skipws); // Disable white-space skipping.
istream_iterator‹char, ptrdiff_t› it1(s); // Position at start of file.
istream_iterator‹char, ptrdiff_t› it2; // Serves as "past-the-end" marker.
copy(it1, it2, back_inserter(buffer));
Line::iterator i = buffer.begin();
Line::iterator p;
while (i != buffer.end()) {
p = find(i, buffer.end(), '\n');
lines.push_back(new Line(i, p));
i = ++p;
}
sort(lines.begin(), lines.end(), less_p‹Line*›());
cout ‹‹ "Read " ‹‹ lines.size() ‹‹ " lines" ‹‹ endl;
vector‹Line*›::iterator j;
for(j = lines.begin(); j!= lines.end(); j++) printLine(*j);
release(lines.begin(), lines.end()); // Release memory.
return 0;
}
alloc1.cpp
#include ‹stl.h›
#include ‹ospace/stl/examples/myaloc.h›
int main() {
{
cout ‹‹ "vectors:" ‹‹ endl;
os_my_allocator‹int› alloc;
vector‹int› v3(alloc);
v3.push_back(42);
vector‹int› v4(alloc);
v4.push_back(42);
}
{
cout ‹‹ "bit_vectors:" ‹‹ endl;
os_my_allocator‹unsigned int› alloc;
bit_vector v1(alloc);
v1.push_back(1);
}
{
cout ‹‹ "deques:" ‹‹ endl;
os_my_allocator‹int› alloc;
deque‹int› d(alloc);
d.push_back(42);
}
{
cout ‹‹ "lists:" ‹‹ endl;
os_my_allocator‹os_list_node‹int› › alloc;
list‹int› l(alloc);
l.push_back(42);
}
{
cout ‹‹ "sets:" ‹‹ endl;
os_my_allocator‹os_value_node‹int› › alloc;
set‹int, less‹int› › s(alloc);
s.insert(42);
}
{
cout ‹‹ "maps" ‹‹ endl;
os_my_allocator‹os_value_node‹os_pair‹const int, float› › › alloc;
map‹int, float, less‹int› › m(alloc);
m[4] = 2.0;
}
return 0;
}
release2.cpp
#include ‹stl.h›
#include ‹iostream.h›
class X {
public:
X(int i_): i (i_) {}
~X() {cout ‹‹ "Delete X(" ‹‹ i ‹‹ ")" ‹‹ endl;}
int i;
};
ostream& operator ‹‹ (ostream& stream_, const X& x_) {
return stream_ ‹‹ "X(" ‹‹ x_.i ‹‹ ")";
}
int main() {
vector‹X*› v;
v.push_back(new X(2));
v.push_back(new X(1));
v.push_back(new X(4));
vector‹X*›::iterator i;
cout ‹‹ "Initial contents:" ‹‹ endl;
for (i = v.begin(); i!= v.end(); i++) cout ‹‹ " " ‹‹ *(*i) ‹‹ endl;
release(v.begin()); // Delete the first heap-based object.
v.erase(v.begin()); // Erase the first element.
cout ‹‹ "Remaining contents:" ‹‹ endl;
for (i = v.begin(); i != v.end(); i++) cout ‹‹ " " ‹‹ *(*i) ‹‹ endl;
release(v.begin(), v.end()); // Delete remaining heap-based objects.
v.erase(v.begin(), v.end()); // Erase remaining elements.
return 0;
}
map1.cpp
#include ‹iostream.h›
#include ‹stl.h›
int main() {
typedef map‹char, int, less‹char› › maptype;
maptype m;
// Store mappings between roman numerals and decimals.
m['l'] = 50;
m['x'] = 20; // Deliberate mistake.
m['v'] = 5;
m['i'] = 1;
cout ‹‹ "m['x'] = " ‹‹ m['x'] ‹‹ endl;
m['x'] = 10; // Correct mistake.
cout ‹‹ "m['x'] = " ‹‹ m['x'] ‹‹ endl;
cout ‹‹ "m['z'] = " ‹‹ m['z'] ‹‹ endl; // Note default value is added.
cout ‹‹ "m.count('z') = " ‹‹ m.count('z') ‹‹ endl;
pair‹maptype::iterator, bool› p;
p = m.insert(pair‹const char, int›('c', 100));
if (p.second) cout ‹‹ "First insertion successful" ‹‹ endl;
p = m.insert(pair‹const char, int› ('c', 100));
if (p.second) cout ‹‹ "Second insertion successful" ‹‹ endl;
else cout ‹‹ "Existing pair " ‹‹ (*(p.first)).first ‹‹ " -› " ‹‹ (*(p.first)).second ‹‹ endl;
return 0;
}
mismtch2.cpp
#include ‹stl.h›
#include ‹iostream.h›
#include ‹string.h›
bool str_equal(const char* a_, const char* b_) {
return ::strcmp(a_, b_) == 0 ? 1: 0;
}
const unsigned size = 5;
char* n1[size] = {"Brett", "Graham", "Jack", "Mike", "Todd"};
int main() {
char* n2[size];
copy(n1, n1 + 5, n2);
pair‹char**, char**› result;
result = mismatch(n1, n1+ size, n2, str_equal);
if (result.first == n1 + size && result.second == n2 + size)
cout ‹‹ "n1 and n2 are the same" ‹‹ endl;
else cout ‹‹ "mismatch at index: " ‹‹ (result.first - n1) ‹‹ endl;
n2[2] = "QED";
result = mismatch(n1, n1 + size, n2, str_equal);
if (result.first == n2 + size && result.second == n2 + size)
cout ‹‹ "n1 and n2 are the same" ‹‹ endl;
else cout ‹‹ "mismatch at index: " ‹‹ (result.first - n1) ‹‹ endl;
return 0;
}
mismtch1.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
typedef vector‹int› IntVec;
IntVec v1(10);
IntVec v2(v1.size());
iota(v1.begin(), v1.end(), 0);
iota(v2.begin(), v2.end(), 0);
pair ‹IntVec::iterator, IntVec::iterator› result;
result = mismatch(v1.begin(), v1.end(), v2.begin());
if (result.first = v1.end() && result.second == v2.end())
cout ‹‹ "v1 and v2 are the same" ‹‹ endl;
else cout ‹‹ "mismatch at index: " ‹‹ (result.first - v1.begin()) ‹‹ endl;
v2[v2.size()/2] = 42;
result = mismatch(v1.begin(), v1.end(), v2.begin());
if (result.first == v1.end() && result.second == v2.end())
cout ‹‹ "v1 and v2 are the same" ‹‹ endl;
else cout ‹‹ "mismatch at index: " ‹‹ (result.first - v1.begin()) ‹‹ endl;
return 0;
}
mmap2.cpp
#include ‹iostream.h›
#include ‹stl.h›
typedef multimap‹int, char, less‹int› › mmap;
typedef pair‹const int, char› pair_type;
pair_type p1(3, 'c');
pair_type p2(6, 'f');
pair_type p3(1, 'a');
pair_type p4(2, 'b');
pair_type p5(3, 'x');
pair_type p6(6, 'f');
pair_type array[] = { p1, p2, p3, p4, p5, p6 };
int main() {
mmap m(array, array + 7);
mmap::iterator i;
// Return location of first element that is not less than 3
i = m.lower_bound(3);
cout ‹‹ "lower bound:" ‹‹ endl;
cout ‹‹ (*i).first ‹‹ " -› " ‹‹ (*i).second ‹‹ endl;
// Return location of first element that is greater than 3
i = m.upper_bound(3);
cout ‹‹ "upper bound:" ‹‹ endl;
cout ‹‹ (*i).first ‹‹ " -› " ‹‹ (*i).second ‹‹ endl;
return 0;
}
adjfind2.cpp
#include ‹stl.h›
#include ‹iostream.h›
#include ‹string.h›
typedef vector‹char*› CStrVector;
int equal_length(const char* v1_, const char* v2_) {
return ::strlen(v1_) == ::strlen(v2_);
}
char* names[] = {"Brett", "Graham", "Jack", "Mike", "Todd"};
int main() {
const int nameCount = sizeof(names)/sizeof(names[0]);
Жалоба
Напишите нам, и мы в срочном порядке примем меры.