Александр Степанов - РУКОВОДСТВО ПО СТАНДАРТНОЙ БИБЛИОТЕКЕ ШАБЛОНОВ (STL) Страница 21
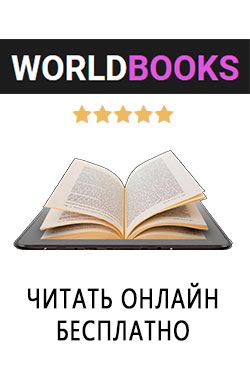
- Категория: Компьютеры и Интернет / Программирование
- Автор: Александр Степанов
- Год выпуска: -
- ISBN: нет данных
- Издательство: -
- Страниц: 30
- Добавлено: 2019-05-29 11:00:25
Александр Степанов - РУКОВОДСТВО ПО СТАНДАРТНОЙ БИБЛИОТЕКЕ ШАБЛОНОВ (STL) краткое содержание
Прочтите описание перед тем, как прочитать онлайн книгу «Александр Степанов - РУКОВОДСТВО ПО СТАНДАРТНОЙ БИБЛИОТЕКЕ ШАБЛОНОВ (STL)» бесплатно полную версию:Александр Степанов - РУКОВОДСТВО ПО СТАНДАРТНОЙ БИБЛИОТЕКЕ ШАБЛОНОВ (STL) читать онлайн бесплатно
int main() {
pair ‹int*, int*› result;
result = mismatch(n1, n1 + 5, n2);
if (result.first == (n1 + 5) && result.second == (n2 + 5))
cout ‹‹ "n1 and n2 are the same" ‹‹ endl;
else cout ‹‹ "Mismatch at offset: " ‹‹ (result.first - n1) ‹‹ endl;
result = mismatch(n1, n1 + 5, n3);
if (result.first == (n1 + 5) && result.second == (n3 + 5))
cout ‹‹ "n1 and n3 are the same" ‹‹ endl;
else cout ‹‹ "Mismatch at offset: " ‹‹ (result.first - n1) ‹‹ endl;
return 0;
}
rndshuf2.cpp
#include ‹stl.h›
#include ‹stdlib.h›
#include ‹iostream.h›
class MyRandomGenerator {
public:
nsigned long operator()(unsigned long n_);
};
unsigned long MyRandomGenerator::operator()(unsigned long n_) {
return rand() % n_;
}
int main() {
vector‹int› v1(10);
iota(v1.begin(), v1.end(), 0);
ostream_iterator ‹int› iter(cout, " ");
copy(v1.begin(), v1.end(), iter);
cout ‹‹ endl;
MyRandomGenerator r;
for (int i = 0; i ‹ 3; i++) {
random_shuffle(v1.begin(), v1.end(), r);
copy(v1.begin(), v1.end(), iter);
cout ‹‹ endl;
}
return 0;
}
merge2.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
vector‹int› v1(5);
vector‹int› v2(v1.size());
for (int i = 0; i ‹ v1.size(); i++) {
v1[i] = 10 - i;
v2[i] = 7 - i;
}
vector‹int› result(v1.size() + v2.size());
merge(v1.begin(), v1.end(), v2.begin(), v2.end(), result.begin(), greater‹int›());
ostream_iterator ‹int› iter(cout, " ");
copy(v1.begin(), v1.end(), iter);
cout ‹‹ endl;
copy(v2.begin(), v2.end(), iter);
cout ‹‹ endl;
copy(result.begin(), result.end(), iter);
cout ‹‹ endl;
return 0;
}
adjfind1.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
typedef vector‹int› IntVector;
IntVector v(10);
for (int i = 0; i ‹ v.size(); i++) v[i] = i;
IntVector::iterator location;
location = adjacent_find(v.begin(), v.end());
if (location != v.end()) cout ‹‹ "Found adjacent pair of: " ‹‹ *location ‹‹ endl;
else cout ‹‹ "No adjacent pairs" ‹‹ endl;
v[6] = 7;
location = adjacent_find(v.begin(), v.end());
if (location!= v.end()) cout ‹‹ "Found adjacent pair of: " ‹‹ *location ‹‹ endl;
else cout ‹‹ "No adjacent pairs" ‹‹ endl;
return 0;
}
vec7.cpp
#include ‹iostream.h›
#include ‹stl.h›
int array1[] = {1, 4, 25};
int array2[] = {9, 16};
int main() {
vector‹int› v(array1, array1 + 3);
v.insert(v.begin(), 0); // Insert before first element.
v.insert(v.end(), 36); // Insert after last element.
for (int i = 0; i ‹ v.size(); i++) cout ‹‹ "v[" ‹‹ i ‹‹ "] = " ‹‹ v[i] ‹‹ endl;
cout ‹‹ endl;
// Insert contents of array2 before fourth element.
v.insert(v.begin() + 3, array2, array2 + 2);
for (i = 0; i ‹ v.size(); i++)
cout ‹‹ "v[" ‹‹ i ‹‹ "] = " ‹‹ v[i] ‹‹ endl;
cout ‹‹ endl;
return 0;
}
bcompos1.cpp
#include ‹iostream.h›
#include ‹stl.h›
struct odd: public unary_function‹int, bool› {
odd() {}
bool operator() (int n_) const {return (n_ % 2) - 1;}
};
struct positive: public unary_function‹int, bool› {
positive() {}
bool operator() (int n_) const {return n_ ›= 0;}
};
int array[6] = {-2, -1, 0, 1, 2, 3};
int main() {
binary_compose‹logical_and‹bool›, odd, positive› b(logical_and‹bool›(), odd(), positive());
int* p = find_if(array, array + 6, b);
if (p != array + 6) cout ‹‹ *p ‹‹ " is odd and positive" ‹‹ endl;
return 0;
}
setsymd2.cpp
#include ‹stl.h›
#include ‹iostream.h›
#include ‹string.h›
char* word1 = "ABCDEFGHIJKLMNO";
char* word2 = "LMNOPQRSTUVWXYZ";
int main() {
ostream_iterator‹char› iter(cout, " ");
cout ‹‹ "word1: ";
copy(word1, word1 + ::strlen(word1), iter);
cout ‹‹ "\nword2: ";
copy(word2, word2 + ::strlen(word2), iter);
cout ‹‹ endl;
set_symmetric_difference(word1, word1 + ::strlen(word1), word2, word2 + ::strlen(word2), iter, less‹char›());
cout ‹‹ endl;
return 0;
}
search0.cpp
#include ‹stl.h›
#include ‹iostream.h›
int v1[6] = {1, 1, 2, 3, 5, 8};
int v2[6] = {0, 1, 2, 3, 4, 5};
int v3[2] = {3, 4};
int main() {
int* location;
location = search(v1, v1 + 6, v3, v3 + 2);
if (location == v1 + 6) cout ‹‹ "v3 not contained in v1" ‹‹ endl;
else cout ‹‹ "Found v3 in v1 at offset: " ‹‹ location - v1 ‹‹ endl;
location = search(v2, v2 + 6, v3, v3 + 2);
if (location == v2 + 6) cout ‹‹ "v3 not contained in v2" ‹‹ endl;
else cout ‹‹ "Found v3 in v2 at offset: " ‹‹ location - v2 ‹‹ endl;
return 0;
}
eqlrnge1.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
typedef vector‹int› IntVec;
IntVec v(10);
for (int i = 0; i ‹ v.size(); i++) v[i] = i / 3; ostream_iterator‹int› iter(cout, " ");
cout ‹‹ "Within the collection:\n\t";
copy(v.begin(), v.end(), iter);
pair‹IntVec::iterator, IntVec::iterator› range;
range = equal_range(v.begin(), v.end(), 2);
cout ‹‹ "\n2 can be inserted from before index " ‹‹ (range.first - v.begin())
‹‹ " to before index " ‹‹ (range.second - v.begin()) ‹‹ endl;
return 0;
}
rotcopy1.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
vector‹int› v1(10);
iota(v1.begin(), v1.end(), 0);
ostream_iterator ‹int› iter(cout, " ");
copy(v1.begin(), v1.end(), iter);
cout ‹‹ endl;
vector‹int› v2(v1.size());
for (int i = 0; i ‹ v1.size(); i++) {
rotate_copy(v1.begin(), v1.begin() + i, v1.end(), v2.begin());
ostream_iterator‹int› iter(cout, " ");
copy(v2.begin(), v2.end(), iter);
cout ‹‹ endl;
}
cout ‹‹ endl;
return 0;
}
eqlrnge2.cpp
#include ‹stl.h›
#include ‹iostream.h›
#include ‹string.h›
char chars[] = "aabbccddggghhklllmqqqqssyyzz";
int main() {
const unsigned count = sizeof(chars) - 1;
ostream_iterator‹char› iter(cout);
cout ‹‹ "Within the collection:\n\t";
copy(chars, chars + count, iter);
pair‹char*, char*› range;
range = equal_range(chars, chars + count, 'q', less‹char›());
cout ‹‹ "\nq can be inserted from before index " ‹‹ (range.first - chars) ‹‹ " to before index "
‹‹ (range.second - chars) ‹‹ endl;
return 0;
}
release1.cpp
#include ‹stl.h›
#include ‹iostream.h›
class X {
public:
X(int i_): i(i_) {}
~X() {cout ‹‹ "Delete X(" ‹‹ i ‹‹ ")" ‹‹ endl;}
int i;
};
ostream& operator ‹‹ (ostream& stream_, const X& x_) {
return stream_ ‹‹ "X(" ‹‹ x_.i ‹‹ ")";
}
int main() {
vector‹X*› v;
v.push_back(new X(2));
v.push_back(new X(1));
v.push_back(new X(4));
vector‹X*›::iterator i;
for (i = v.begin(); i!= v.end(); i++) cout ‹‹ *(*i) ‹‹ endl;
release(v.begin(), v.end()); // Delete heap-based objects.
return 0;
}
incl1.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
vector‹int› v1(10);
vector‹int› v2(3);
for (int i = 0; i ‹ v1.size(); i++) {
v1[i] = i;
}
if (includes(v1.begin(), v1.end(), v2.begin(), v2.end())) cout ‹‹ "v1 includes v2" ‹‹ endl;
else cout ‹‹ "v1 does not include v2" ‹‹ endl;
for (i = 0; i ‹ v2.size(); i++) v2[i] = i + 3;
if (includes(v1.begin(), v1.end(), v2.begin(), v2.end())) cout ‹‹ "v1 includes v2" ‹‹ endl;
else cout ‹‹ "v1 does not include v2" ‹‹ endl;
return 0;
}
setintr2.cpp
#include ‹stl.h›
#include ‹iostream.h›
#include ‹string.h›
char* word1 = "ABCDEFGHIJKLMNO";
char* word2 = "LMNOPQRSTUVWXYZ";
int main() {
ostream_iterator ‹char› iter(cout, " ");
cout ‹‹ "word1: ";
copy(word1, word1 + ::strlen(word1), iter);
cout ‹‹ "\nword2: ";
copy(word2, word2 + ::strlen(word2), iter);
cout ‹‹ endl;
set_intersection(word1, word1 + ::strlen(word1), word2, word2 + ::strlen(word2), iter, less‹char›());
cout ‹‹ endl;
return 0;
}
inrprod1.cpp
#include ‹stl.h›
#include ‹iostream.h›
#include ‹string.h›
int main() {
vector‹int› v1(3);
vector‹int› v2(v1.size());
for (int i = 0; i ‹ v1.size(); i++) {
v1[i] = i + 1;
v2[i] = v1.size() - i;
}
ostream_iterator‹int› iter(cout, " ");
cout ‹‹ "Inner product(sum of products) of:\n\t";
copy(v1.begin(), v1.end(), iter);
cout ‹‹ "\n\t";
copy(v2.begin(), v2.end(), iter);
int result = inner_product(v1.begin(), v1.end(), v2.begin(), 0);
Жалоба
Напишите нам, и мы в срочном порядке примем меры.