Александр Степанов - РУКОВОДСТВО ПО СТАНДАРТНОЙ БИБЛИОТЕКЕ ШАБЛОНОВ (STL) Страница 30
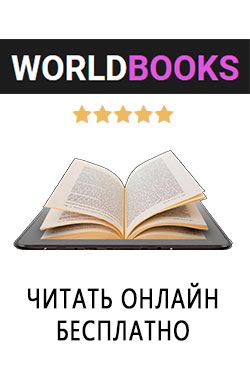
- Категория: Компьютеры и Интернет / Программирование
- Автор: Александр Степанов
- Год выпуска: -
- ISBN: нет данных
- Издательство: -
- Страниц: 30
- Добавлено: 2019-05-29 11:00:25
Александр Степанов - РУКОВОДСТВО ПО СТАНДАРТНОЙ БИБЛИОТЕКЕ ШАБЛОНОВ (STL) краткое содержание
Прочтите описание перед тем, как прочитать онлайн книгу «Александр Степанов - РУКОВОДСТВО ПО СТАНДАРТНОЙ БИБЛИОТЕКЕ ШАБЛОНОВ (STL)» бесплатно полную версию:Александр Степанов - РУКОВОДСТВО ПО СТАНДАРТНОЙ БИБЛИОТЕКЕ ШАБЛОНОВ (STL) читать онлайн бесплатно
q.pop();
}
return 0;
}
stack1.cpp
#include ‹iostream.h›
#include ‹stl.h›
int main() {
stack‹deque‹int› › s;
s.push(42);
s.push(101);
s.push(69);
while (!s.empty()) {
cout ‹‹ s.top() ‹‹ endl;
s.pop();
}
return 0;
}
greateq.cpp
#include ‹iostream.h›
#include ‹stl.h›
int array[4] = {3, 1, 4, 2};
int main() {
sort(array, array + 4, greater_equal‹int›());
for (int i = 0; i ‹ 4; i++) cout ‹‹ array[i] ‹‹ endl;
return 0;
}
stack2.cpp
#include ‹iostream.h›
#include ‹stl.h›
int main() {
stack‹list‹int› › s;
s.push(42);
s.push(101);
s.push(69);
while (!s.empty()) {
cout ‹‹ s.top() ‹‹ endl;
s.pop();
}
return 0;
}
lesseq.cpp
#include ‹iostream.h›
#include ‹stl.h›
int array[4] = {3, 1, 4, 2};
int main() {
sort(array, array + 4, less_equal‹int›());
for (int i = 0; i ‹ 4; i++) cout ‹‹ array[i] ‹‹ endl;
return 0;
}
divides.cpp
#include ‹iostream.h›
#include ‹stl.h›
int input[3] = {2, 3, 4};
int main() {
int result = accumulate(input, input + 3, 48, divides‹int›());
cout ‹‹ "result = " ‹‹ result ‹‹ endl;
return 0;
}
greater.cpp
#include ‹iostream.h›
#include ‹stl.h›
int array[4] = {3, 1, 4, 2};
int main() {
sort(array, array + 4, greater‹int›());
for (int i = 0; i ‹ 4; i++) cout ‹‹ array[i] ‹‹ endl;
return 0;
}
swap1.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
int a = 42;
int b = 19;
cout ‹‹ "a = " ‹‹ a ‹‹ " b = " ‹‹ b ‹‹ endl;
swap(a, b);
cout ‹‹ "a = " ‹‹ a ‹‹ " b = " ‹‹ b ‹‹ endl;
return 0;
}
times.cpp
#include ‹iostream.h›
#include ‹stl.h›
int input[4] = {1, 5, 7, 2};
int main() {
int total = accumulate(input, input + 4, 1, times‹int›());
cout ‹‹ "total = " ‹‹ total ‹‹ endl;
return 0;
}
less.cpp
#include ‹iostream.h›
#include ‹stl.h›
int array[4] = {3, 1, 4, 2};
int main() {
sort(array, array + 4, less‹int› ());
for (int i = 0; i ‹ 4; i++) cout ‹‹ array[i] ‹‹ endl;
return 0;
}
alg1.cpp
#include ‹iostream.h›
#include ‹stl.h›
int main() {
int i = min(4, 7);
cout ‹‹ "min(4, 7) = " ‹‹ i ‹‹ endl;
char c = maX('a', 'z');
cout ‹‹ "maX('a', 'z') = " ‹‹ c ‹‹ endl;
return 0;
}
filln1.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
vector‹int› v(10);
fill_n(v.begin(), v.size(), 42);
for (int i = 0; i ‹ 10; i++) cout ‹‹ v[i] ‹‹ ' ';
cout ‹‹ endl;
return 0;
}
iota1.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
int numbers[10];
iota(numbers, numbers + 10, 42);
for (int i = 0; i ‹ 10; i++) cout ‹‹ numbers[i] ‹‹ ' ';
cout ‹‹ endl;
return 0;
}
nextprm0.cpp
#include ‹stl.h›
#include ‹iostream.h›
int v1[3] = {0, 1, 2};
int main() {
next_permutation(v1, v1 + 3);
for (int i = 0; i ‹ 3; i++) cout ‹‹ v1[i] ‹‹ ' ';
cout ‹‹ endl;
return 0;
}
prevprm0.cpp
#include ‹stl.h›
#include ‹iostream.h›
int v1[3] = {0, 1, 2};
int main() {
prev_permutation(v1, v1 + 3);
for (int i = 0; i ‹ 3; i++) cout ‹‹ v1[i] ‹‹ ' ';
cout ‹‹ endl;
return 0;
}
fill1.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
vector‹int› v(10);
fill(v.begin(), v.end(), 42);
for (int i = 0; i ‹ 10; i++) cout ‹‹ v[i] ‹‹ ' ';
cout ‹‹ endl;
return 0;
}
pair2.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
pair‹int, int› p = make_pair(1, 10);
cout ‹‹ "p.first = " ‹‹ p.first ‹‹ endl;
cout ‹‹ "p.second = " ‹‹ p.second ‹‹ endl;
return 0;
}
error1.cpp
#include ‹stl.h›
// Compile this code without defining the symbol OS_USE_EXCEPTIONS.
int main() {
vector‹int› v;
v.pop_back(); // Generates an empty object error.
return 0;
}
pair0.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
pair‹int, int› p = make_pair(1, 10);
cout ‹‹ "p.first = " ‹‹ p.first ‹‹ ", p.second = " ‹‹ p.second ‹‹ endl;
return 0;
}
pair1.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
pair‹int, int› p = make_pair(1, 10);
cout ‹‹ "p.first = " ‹‹ p.first ‹‹ ", p.second = " ‹‹ p.second ‹‹ endl;
return 0;
}
minelem1.cpp
#include ‹stl.h›
#include ‹iostream.h›
int numbers[6] = {-10, 15, -100, 36, -242, 42};
int main() {
cout ‹‹ *min_element(numbers, numbers + 6) ‹‹ endl;
return 0;
}
maxelem1.cpp
#include ‹stl.h›
#include ‹iostream.h›
int numbers[6] = {4, 10, 56, 11, -42, 19};
int main() {
cout ‹‹ *max_element(numbers, numbers + 6) ‹‹ endl;
return 0;
}
max1.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
cout ‹‹ max(42, 100) ‹‹ endl;
return 0;
}
min1.cpp
#include ‹stl.h›
#include ‹iostream.h›
int main() {
cout ‹‹ min(42, 100) ‹‹ endl;
return 0;
}
adjdiff0.cpp
#include ‹stl.h›
#include ‹iostream.h›
int numbers[5] = {1, 2, 4, 8, 16};
int main() {
int difference[5];
adjacent_difference(numbers, numbers + 5, difference);
for (int i = 0; i ‹ 5; i++) cout ‹‹ numbers[i] ‹‹ ' ';
cout ‹‹ endl;
for (i = 0; i ‹ 5; i++) cout ‹‹ difference[i] ‹‹ ' ';
cout ‹‹ endl;
return 0;
}
Жалоба
Напишите нам, и мы в срочном порядке примем меры.